How to use setTimeout with Vue | Equivalent of setTimeout() function.
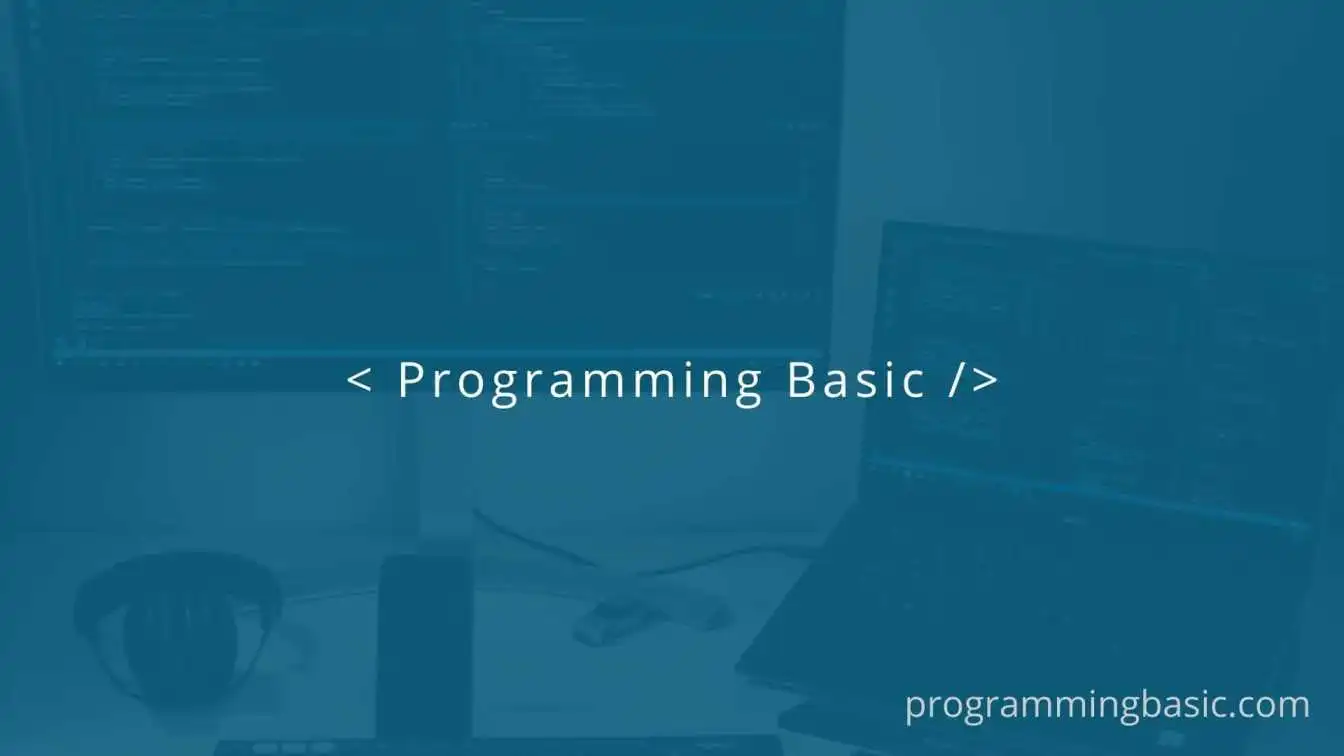
In this short post, we will see how to use the setTimeout() function in Vue Js.
The setTimeout()
function method executes a function or a block of code after a specific time.
Syntax:
setTimeout(function, milliseconds);
Here, 1000 miliseconds = 1second
The setTimeout
is useful to run a function after a specified time delay or sometimes we can use it to emulate a server request for some demos.
Now let's see how we can use the setTimeout()
in a Vue method to change the text of a variable.
<template>
<div id="app">
<button @click="changeText()">Change Text</button>
<h1>{{ text }}</h1>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
text: "Hello World",
};
},
methods: {
changeText() {
setTimeout(() => {
this.text = "Welcome World";
}, 2000);
},
},
};
</script>
Here, we have a function changeText()
, which have a setTimeout() function that change the text after 2 seconds.
So now when we click the button, it executes the changeText()
method, and the this.text
changes to 'Welcome World' after a delay of 2 seconds.
Code Demo: