JavaScript - Convert Days to Seconds
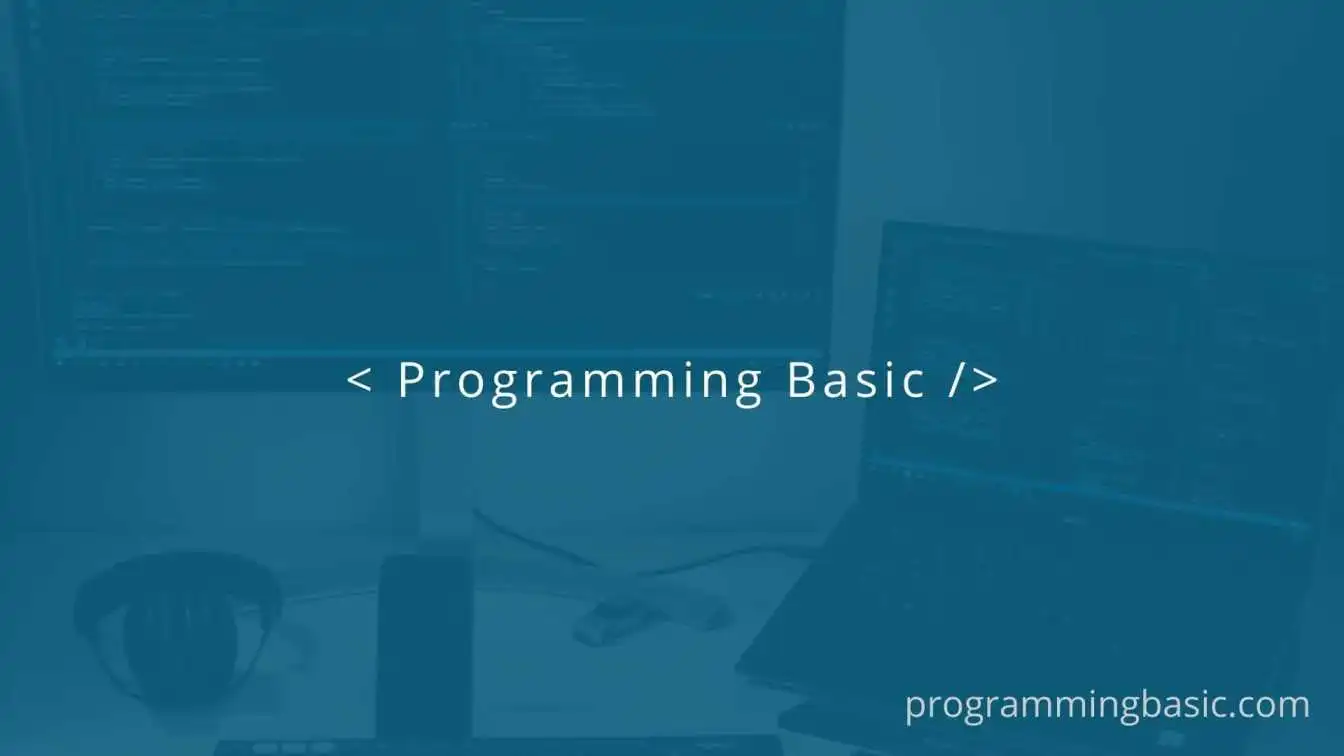
In this tutorial, we will see how to convert days to seconds using JavaScript.
Before writing the program we should know that:
- There are 24 hours in one day
- 60 minutes in an hour, and
- 60 seconds in a minute
So, to convert days into seconds we have to multiply the days by 24 to get hours, 60 for the minutes and 60 for the seconds.
seconds = days * 24 * 60 * 60
Let's see it in code
const daysToSec = (days) => {
return days * 24 * 60 * 60;
}
console.log(daysToSec(1)) //86400
console.log(daysToSec(20)) //1728000
Here, we have created a function daysToSec() , which take days as an argument and converts the days into seconds.
If you input your days in decimal form, it will return the seconds in decimal.
const daysToSec = (days) => {
return days * 24 * 60 * 60;
}
console.log(daysToSec(1.534) + ' sec') //132537.6 sec
To solve this issue we have to use the Math.round() function of JavaScript.
The Math.round() function round a number to the nearest integer in JavaScript. For example,
Math.round(2.60); // 3
Math.round(4.50); // 5
Math.round(5.49); // 5
So we can modify the above daysToSec()
function to round up the value.
const daysToSec = (days) => {
return Math.round(days * 24 * 60 * 60);
}
console.log(daysToSec(1.534) + ' sec') //132538 sec
Conclusion: To convert days to seconds uisng JavaScript, we have to multiply the days by 24 hours, 60 minutes and 60 seconds i.e days*24*60*60
.
Related Articles:
Convert seconds to minutes and seconds (mm:ss) using JavaScript