How to Remove Last Character from a string in javascript
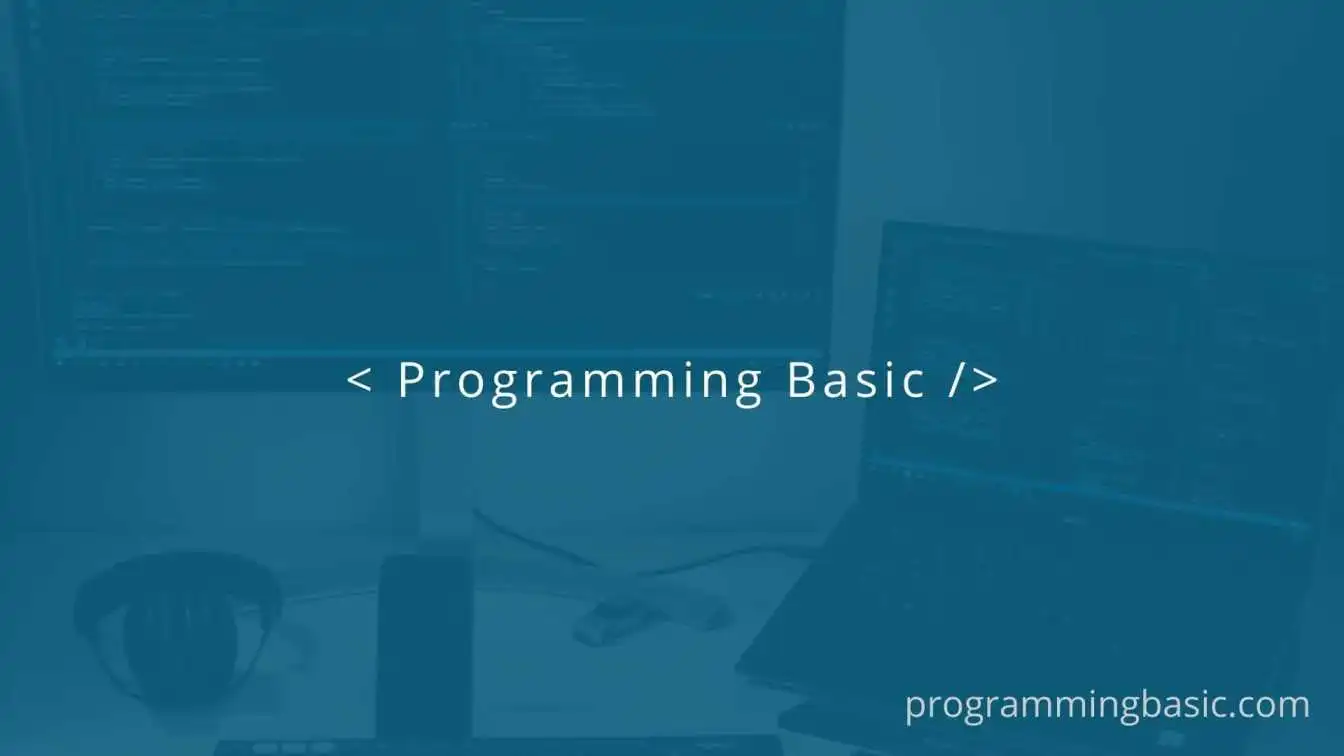
📋 Table Of Content
In this article, we will learn how to remove last character from a string in JavaScript.
Here we will be using two in-built JavaScript methods to remove the last character from a string easily.
We will be using slice()
and substring()
method to slice or trim off the last character from a string.
Method 1 : Using slice() in JavaScript
The slice() method helps us to remove or trim off a character from a string at a specific index. It do not change or modify the original string.
Syntax:
String.slice(start,end)
I have a start index and end index to specific which part of the string you want to extract.
Example:
var str = "Hello World";
var newStr = str.slice(0, -1);
console.log(newStr)
Output:
Hello Worl
Here the starting value is 0 and the end is -1
, because a negative index indicate an offset from the end of the string.
The negative index let us to extract / remove character from the end of the string.
If you give -2
, it will remove the last two characters from the end of the given string.
Method 2 : Using substring() function
The substring()
method in JavaScript remove the characters between two given points: startIndex and endIndex(excluding) and return us a sub-string.
It does not change or modify the original string.
Syntax:
String.substring(indexStart, indexEnd)
IMPORTANT : The character in the startIndex is always included and the character in the endIndex is excluded while extracting the string.
Example:
var str = "Hello World";
var newStr = str.substring(0, str.length-1);
console.log(newStr)
Output:
Hello Worl
Here the start index is 0
and the end index is str.length-1
, and anything between these two points is the extracted string.
Related Topics: