Convert a Unix timestamp to time in JavaScript
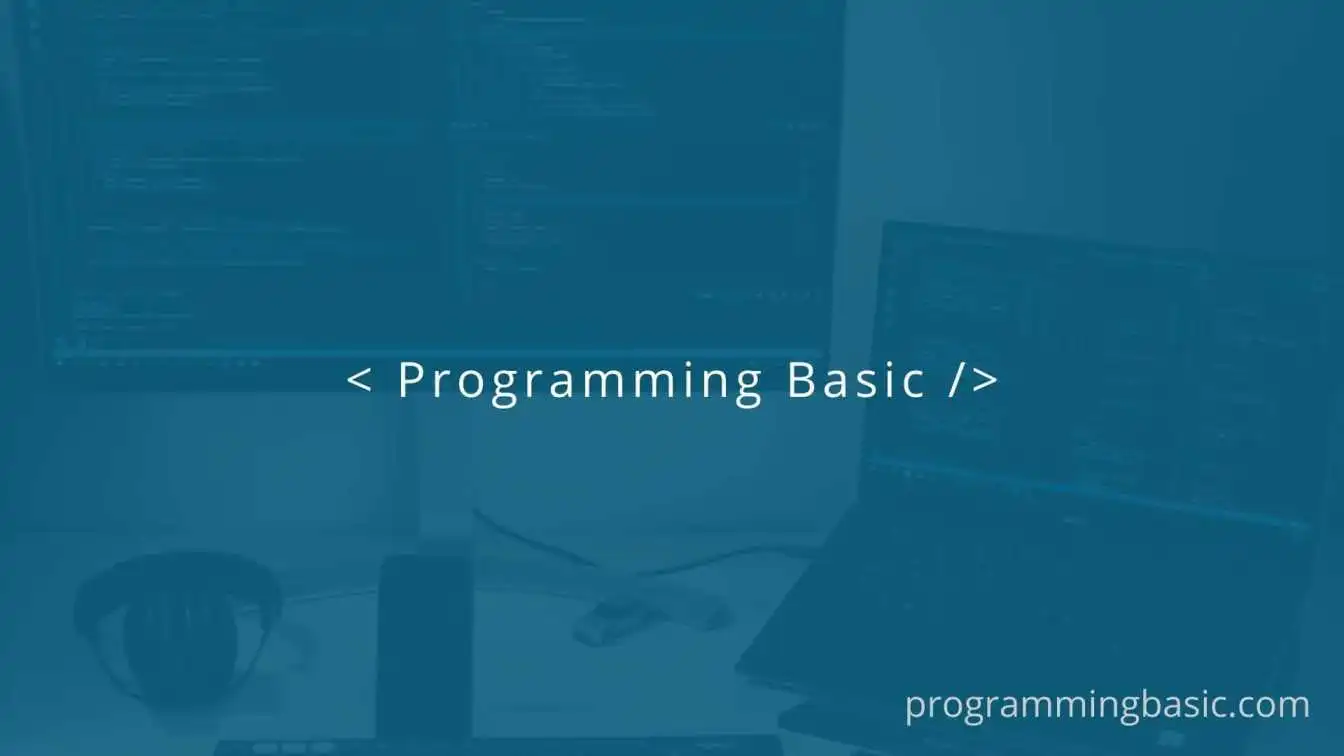
📋 Table Of Content
This short out how to convert a Unix timestamp to a time format using JavaScript.
The UNIX time (also known as Epoch time or UNIX Epoch time) is a single signed number that increases every second. The timestamp of UNIX time is the total number of seconds that's been calculated since January 1, 1970 (midnight UTC/GMT).
The timestamp makes it easier for the computer to manipulate or store than the conventional date-time formats.
So to convert a UNIX timestamp to a time format in JavaScript, we can follow these steps:
- Convert Unix timestamp from seconds to milliseconds by multiplying it by 1000.
- Create a new date object using the
new Date()
constructor method. - Use
toLocaleTimeString()
method to extract the time.
Convert Unix timestamp to time using toLocaleTimeString()
let unix_timestamp = 1549312452
var date = new Date(unix_timestamp * 1000);
console.log(date.toLocaleTimeString())
Output:
8:34:12 PM
Code Explanation:
unix_timestamp 1000* : So that the argument is in milliseconds instead of seconds
date.toLocaleTimeString() : It returns us the time portion of the date as a string using locale conventions.
If you want to remove the seconds ( like this, 8:34 PM ) from to toLocaleTimeString() method, you can code like this.
console.log(date.toLocaleTimeString([], {timeStyle: 'short'}))
// Output : 8:34 PM
The timeStyle is the time formatting style that can be used as an optional argument. It gives us 4 options: full, long, medium, short.
Resources:
Date.prototype.toLocaleTimeString()
Related Topics: