How to convert a decimal number to an integer in Vue
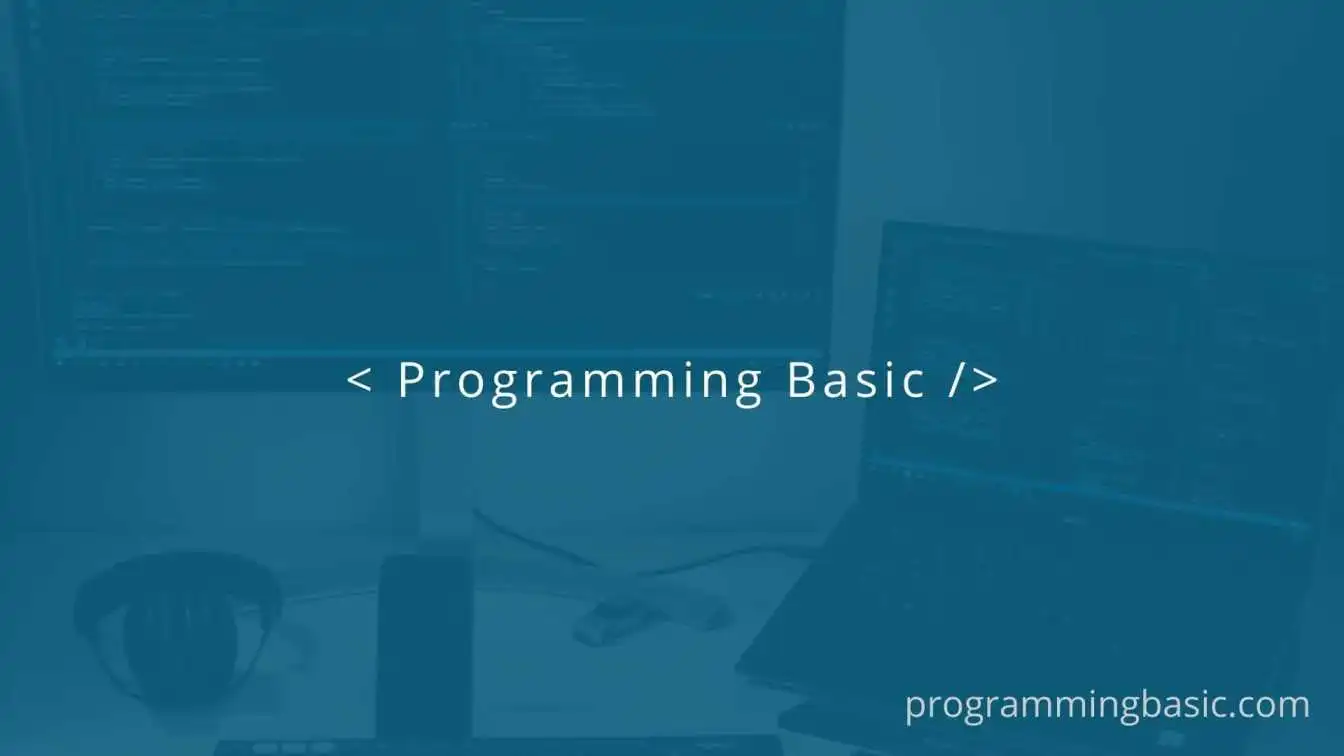
In this short tutorial, we will see how to convert a decimal number to an integer in Vue.
If we generate a random number using Math.random()
function we get random decimal numbers like this :
Random number: 46.32594933788556
Now if we want to convert these values in our vue template to an integer (eg 46), then we can use the parseInt()
method.
The parseInt()
method analyzes a character string that is passed as an argument and converts it to an integer value.
Syntax :
parseInt(string);
So, using this method we can convert the number to an integer by passing the decimal number in parseInt() as an argument in our Vue template.
<template>
<div id="app">
<div>Random number: {{ parseInt(randomNumber) }}</div>
</div>
</template>
Full code to convert the random value to an integer:
<template>
<div id="app">
<div>Random number: {{ parseInt(randomNumber) }}</div>
<button @click="generateNumber()">Generate Number</button>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
randomNumber: "",
};
},
methods: {
generateNumber() {
this.randomNumber = Math.random() * 100; //multiply to generate random number between 0, 100
console.log(typeof this.randomNumber);
},
},
};
</script>