How to check if String contains only spaces in JavaScript
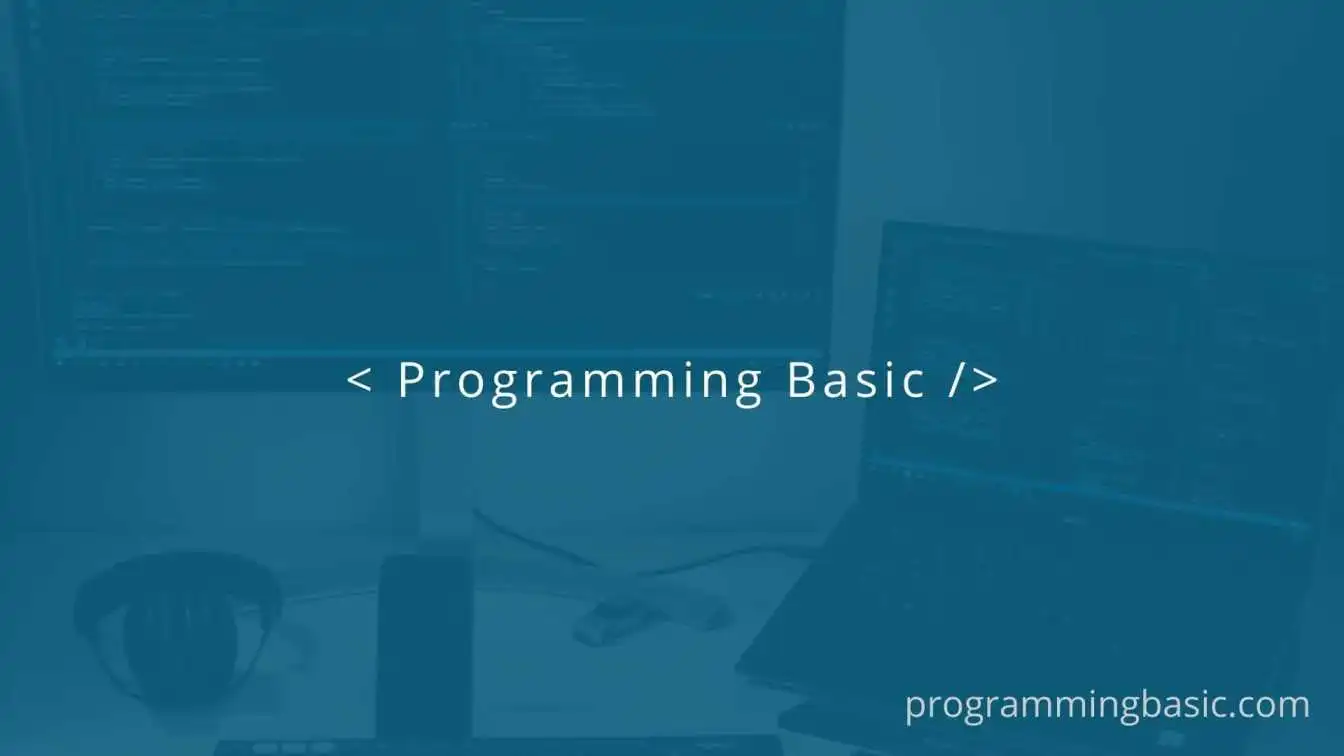
📋 Table Of Content
In this article, we will see how to check if a string is empty or contains only spaces in JavaScript.
Here, we will create a JavaScript function that will check strings with only spaces using :
- trim() method, and
- Regular expressions and test() method
Let's see each method with an example.
Check if a string contains only spaces using trim() method.
The trim()
method in Javascript removes whitespaces from both sides of a string and returns a new string without modifying the original string.
With trim() and the length property, we can determine if a string is empty or have only spaces in it.
This will give us a result in boolean. If the string has only space it will return true
or else it will return false
.
Example:
function checkOnlySpace(str) {
return str.trim().length === 0;
}
console.log(checkOnlySpace(' ')) // true
console.log(checkOnlySpace('')) // true
console.log(checkOnlySpace(' 123 ')) // false
In the above code,
The str.trim()
removes all the whitespaces from both ends of a given string. And using the length property we check if the string contains any character.
If the string does not contain any character, str.trim().length
will return 0 .
Check if a string contains only space using regular expression and test() method
We can use RegExp.test() along with regular expression to check if a string contains only spaces.
The test()
method executes a search for a match in a string. It returns true
if a match is found, otherwise, it returns false
.
Syntax:
RegExp.test(string)
Let's see an example using test() and regular expression to check for strings with only spaces or empty.
function checkOnlySpace(str) {
return /^\s*$/.test(str);
}
console.log(checkOnlySpace(' ')) // true
console.log(checkOnlySpace('')) //true
console.log(checkOnlySpace(' 123 ')) //false
The / /
marks the start and end of a regular expression.
The ^
sign matches the beginning of the input and $
matches the end of the input.
The \s
is used to match any single space, tabs, or newline.
The *
(asterisk) matches the preceding item "\s" 0 or more times in the string.