Check if a HTML checkbox is checked using JavaScript
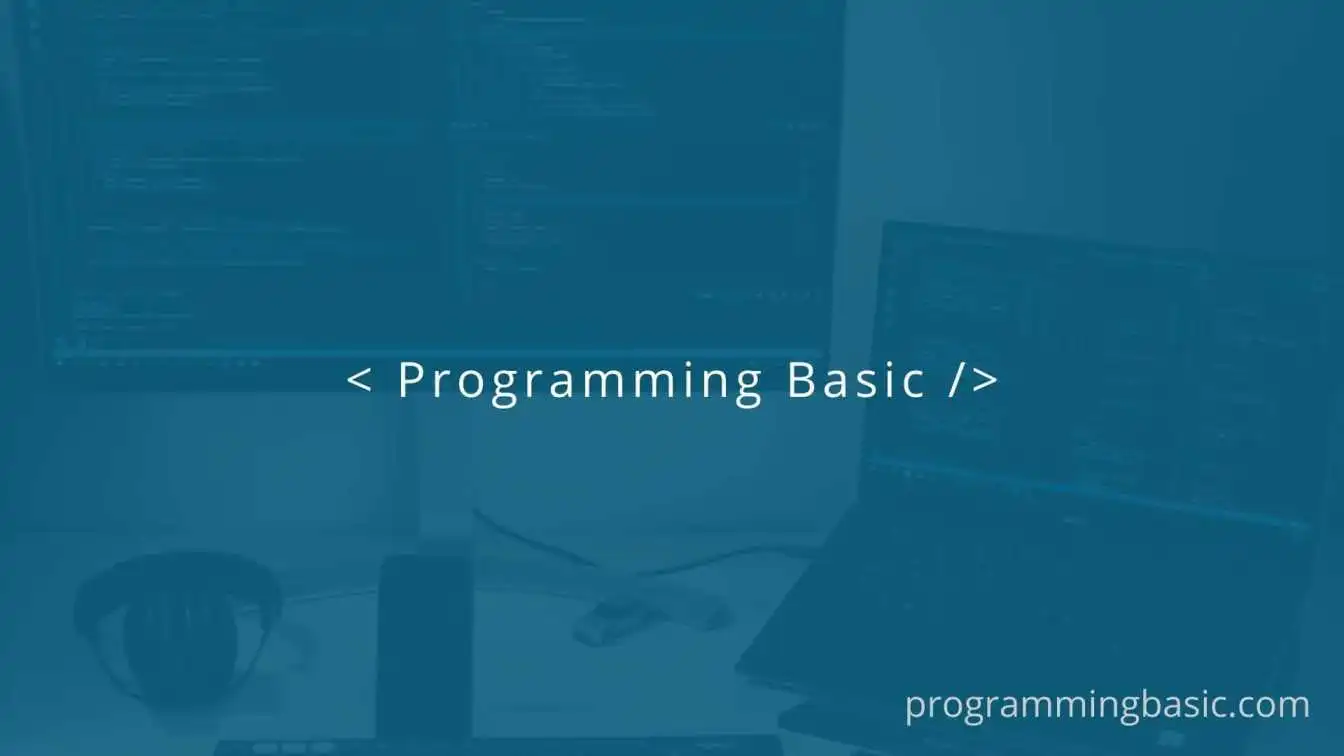
If you are looking for "How do I check if a checkbox is checked in JavaScript?"
The simple answer is using checked
property in JavaScript.
The checked is a boolean property that will return true
if the checkbox is clicked and checked or else it will return false
.
Let us see an example of how to use checked property in JavaScript.
First, let us make a checkbox in HTML with a label.
<input type="checkbox" id="checkedbox" />
<label for="">Agree to Terms and Conditions</label>
and we will have a Button with an id as "btn".
<button id="btn">Submit</button>
And now we will write a JavaScript code where when the button is clicked , it will check if the checkbox in the HTML is checked or not.
If it's checked it will alert as "Checked" or else it will alert "Not Checked".
HTML Code :
<form>
<input type="checkbox" id="checkedbox" />
<label>Agree to Terms and Conditions</label>
<div>
<button id="btn">Submit</button>
</div>
</form>
Script:
const btn = document.querySelector("#btn");
btn.addEventListener("click", (e) => {
e.preventDefault();
validate();
});
function validate() {
var checkedbox = document.getElementById("checkedbox");
if (checkedbox.checked) {
alert("Checked");
} else {
alert("Not Checked");
}
}
In the script code, we have a function called validate(), which will run when we click the submit button.
Since checked is a boolean property, we can use it in any if..else
condition. If the condition is true
, it will alert "Checked", if not it will alert "Not Checked"
Code Demo:
Related Topics:
Change Background Color Using JavaScript